- Published on
How to Use RobotFramework with Examples
- Authors
- Name
- Adam Piskorek
- @hvitis_
Core of Robot Framework
This is not Python addon! I know you might have heard a lot of times that Robot and Python go together but it is only partially true.
It is correct that you can use Python with Robot Framework to create custom scripts but you can use Java as well.
If you think you can be fluent in Robot Framework immediately just by knowing Python then you are wrong. You need to master a couple of Robot syntax before using it.
In order to write test cases you will need to work hand in hand with documentation, especially Selenium (if you want to test frontend) in order to master the "spoke like" syntax.
Examples are:
- Using 3 asterisks around section title in order to write section-specific code:
*** Keywords ***
or
*** Variables ***
or
*** Tasks ***
or
*** Test Cases ***
- Creating custom keywords and using Gherkin BDD syntax:
Test mosaic maker using bdd syntax
Given The Mosaic Maker Is Open
When The User Enters New Image
And The BoardSize is Selected to 3
Then The Result Should Be Minimum 500px wide
- Mixing variables
There is a possibility to use variables that look like JavaScript one:
Set Test Variable ${term}
and it is possible to pass variables to test cases e.g. by importing:
*** Settings ***
Resource ../../../../testdata/variables/imports.resource
Robot Syntax
Most of the implemented, out of the box, ready to use syntax is plain English language. There are some things that should be learned such as:
- What are invisible and superimportant parts of syntax?
- How to use keywords?
- How to define variables (special characters)?
- How to use variables?
Let's answer then now:
Spaces. You might find it weird and a bit pythonic, but one space is not enough to delimiter your variable from it's value. You need more spaced or tab.
Key idea is to write keywords (which are like functions in programming languages) and after that write parameters divided by tabs or many spaces.
Comments - # like in python
Keywor__S__! This is a reminder that we are talking about multiple words. In order to use keywords you should use plain english to define one. You can think of then as of functions in other languages rather than as of variables. A single keybword can be composed out of many english words but in order to reuse it - treat it as a phrase.
In this example the keyword is a whole sentence: open the site and it does what it says. Down below we specify the steps that the keyword will be responsible for.
*** Keywords ***
open the site
Open Browser www.google.pl chrome
We reuse keywords like that:
*** Test Case ***
Website defaults to english language
open the site
Element Text Should Be id:title Welcome
Setting up Docker
In order to quickly start learning by doing let us execute the tests in the best was possible which is of course with Docker. We are going to be using Docker image that has Robot Framework with additional libraries such as Selenium. This was we should worry only about our tests. Later on Docker should enable you also easy CICD implementation.
I am going to use this Docker image (you might need a docker account to see it). You can create your own by reading official tutorial.
Let's create a simple docker compose file:
services:
robot:
image: ppodgorsek/robot-framework:latest
container_name: robot
restart: always
user: root
volumes:
- ./tests:/tests
environment:
WORKSPACE: '/tests:/tests'
BROWSER: 'chrome'
ROBOT_TESTS_DIR: "$WORKSPACE/tests/robot/tests/e2e/"
ROBOT_REPORTS_DIR: "$WORKSPACE/tests/reports/robot/"
network_mode: "host"
extra_hosts:
- "host.docker.internal:host-gateway"
This docker-compose.yml file assumes the following:
- That this file is within a folder with tests catalog
- That you will be running an app on localhost
If you want this service to be a part of docker compose that has backend or frontend service that you want to test, simply delete the network_mode and add shared network.
networks:
- sharednetwork
networks:
sharednetwork:
Now in the folder /tests/robot/tests/e2e/ we will place a file called login.robot
*** Test Cases ***
Verify setup
Log To Console The docker executes my tests!
Now after running command:
docker compose up
You should see something like this:
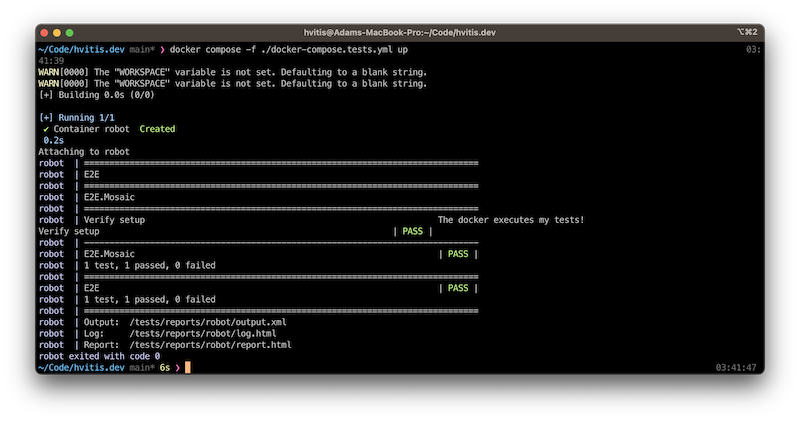
Robot Framework Usage and Examples
Now we can add on to the previous crude example:
*** Settings ***
Library SeleniumLibrary
*** Variables ***
${BROWSER} Chrome
${URL} https://ADDRESS_TO_YOUR_TEST_PAGE
*** Test Cases ***
First test
Log To Console Opening the browser.
Open Browser ${URL} ${BROWSER}
Close Browser
Within the docker there is Robot Framework installed but it requires additional library to work with front end tests. We will check if the Selenium addon that is responsible for that works fine. If so, we will proceed to the actual test.
You are reading this now on my website, which has also a LEGO mosaic projekt.
You can create mosaic there but we will use it now to prepare some test cases that can verify some of the functionalities.
Because we will be creating multiple tests, we should begin each one with the same sequence of events. In our case open webpage at a specific address and make sure it loads correctly.
In order to achieve this we will create a keywords:
*** Keywords ***
Open Mosaic Page
Open Browser ${URL} ${BROWSER}
Wait Until Element Is Visible id:title
This will allow us to write a following test cases (they all begin with the same keyword):
*** Test Cases ***
Page opens correctly
Open Mosaic Page
Input changer induces slider change
Open Mosaic Page
Input Text id:board-size 15
Element Text Should Be id:board-size-text width 10 x height 105
Notification can be closed
Element Text Should Be id:notification-title New version has arrived!
Click Button id:notification-close
Element Should Not Be Visible id:notification-title
Language can be switched to English
Open Mosaic Page
Click Button id:language-switch
Element Text Should Be id:title Mosaic Art Maker
Change color set
Open Mosaic Page
Click Button id:select-set
Click select-31199
# Assess that number of colours decreased
${count} = Get Element Count id:colors-selected
Should Be True ${count} < 30
Those tests should give the following outcome:
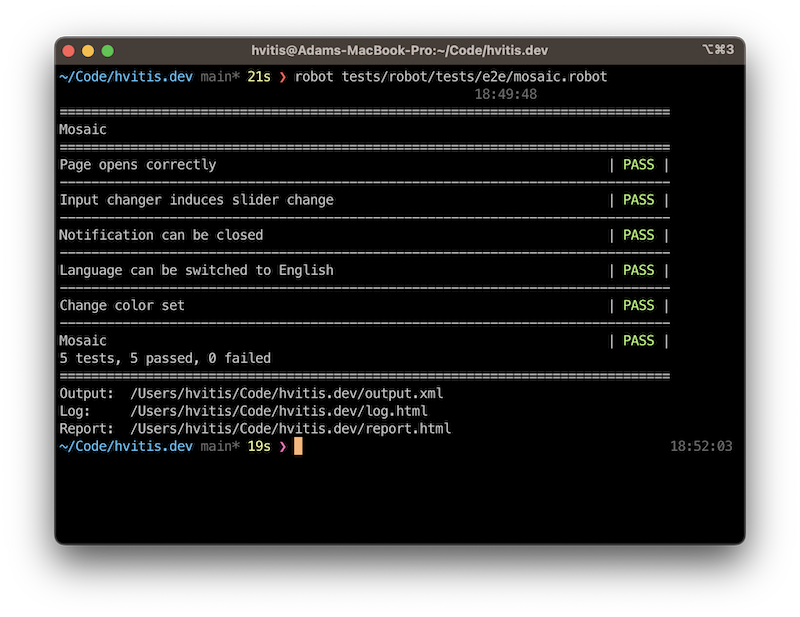
And we also get a HTML report immediately:
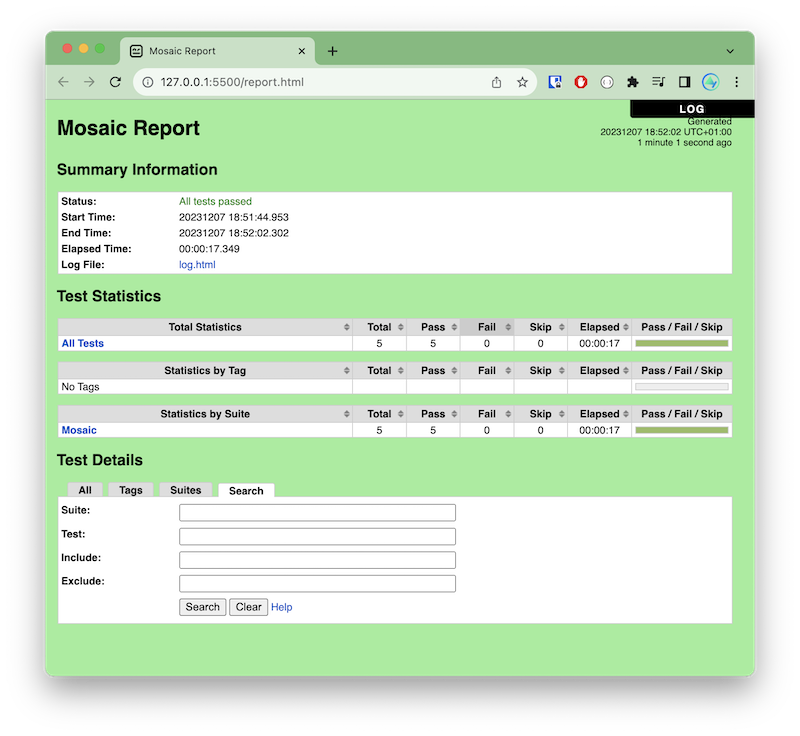
You can see that there was... not much Python. Pretty much the most Pythonic way so far was the english-like syntax, comments and indents.
More to come in the next part...!